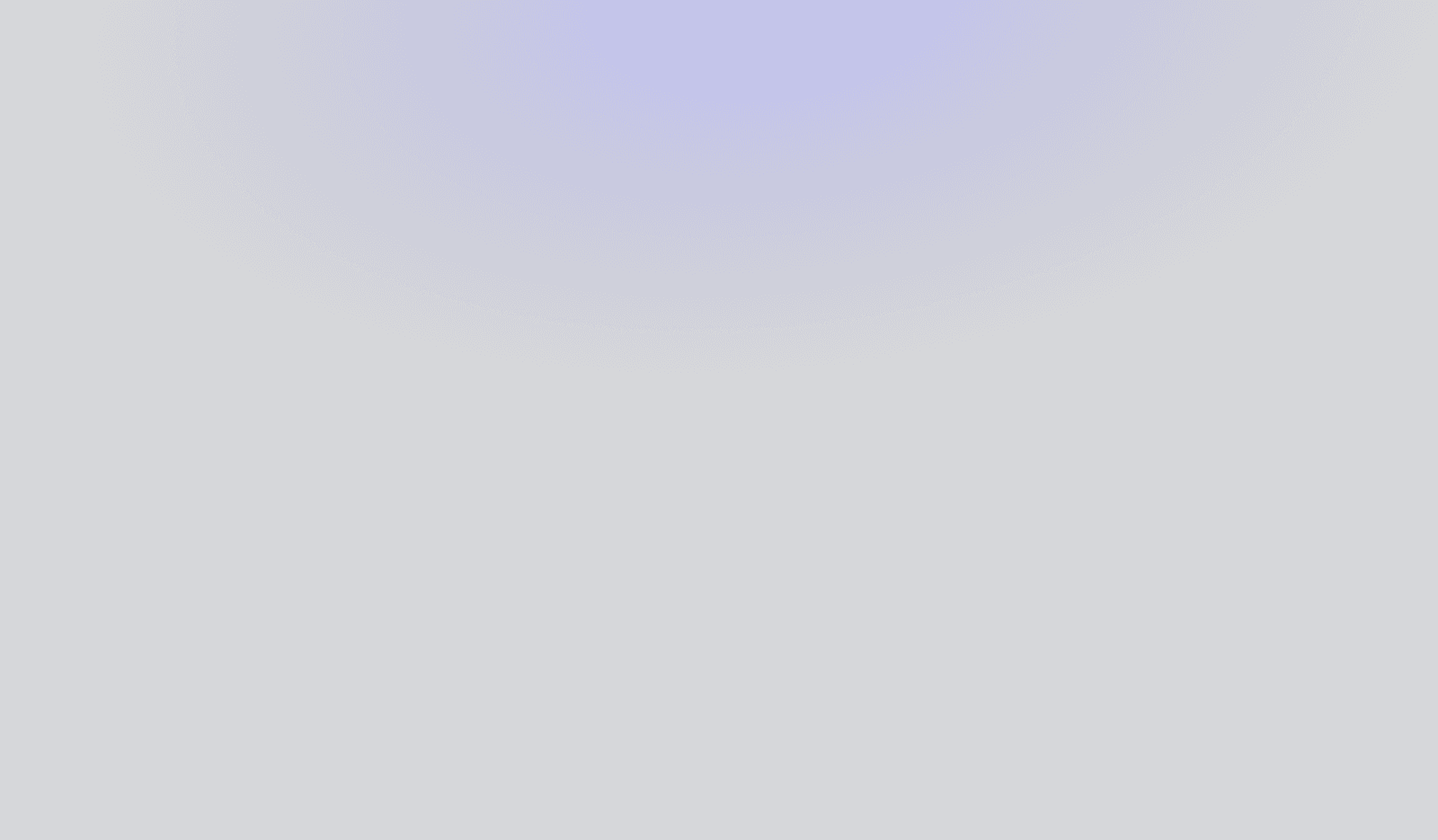
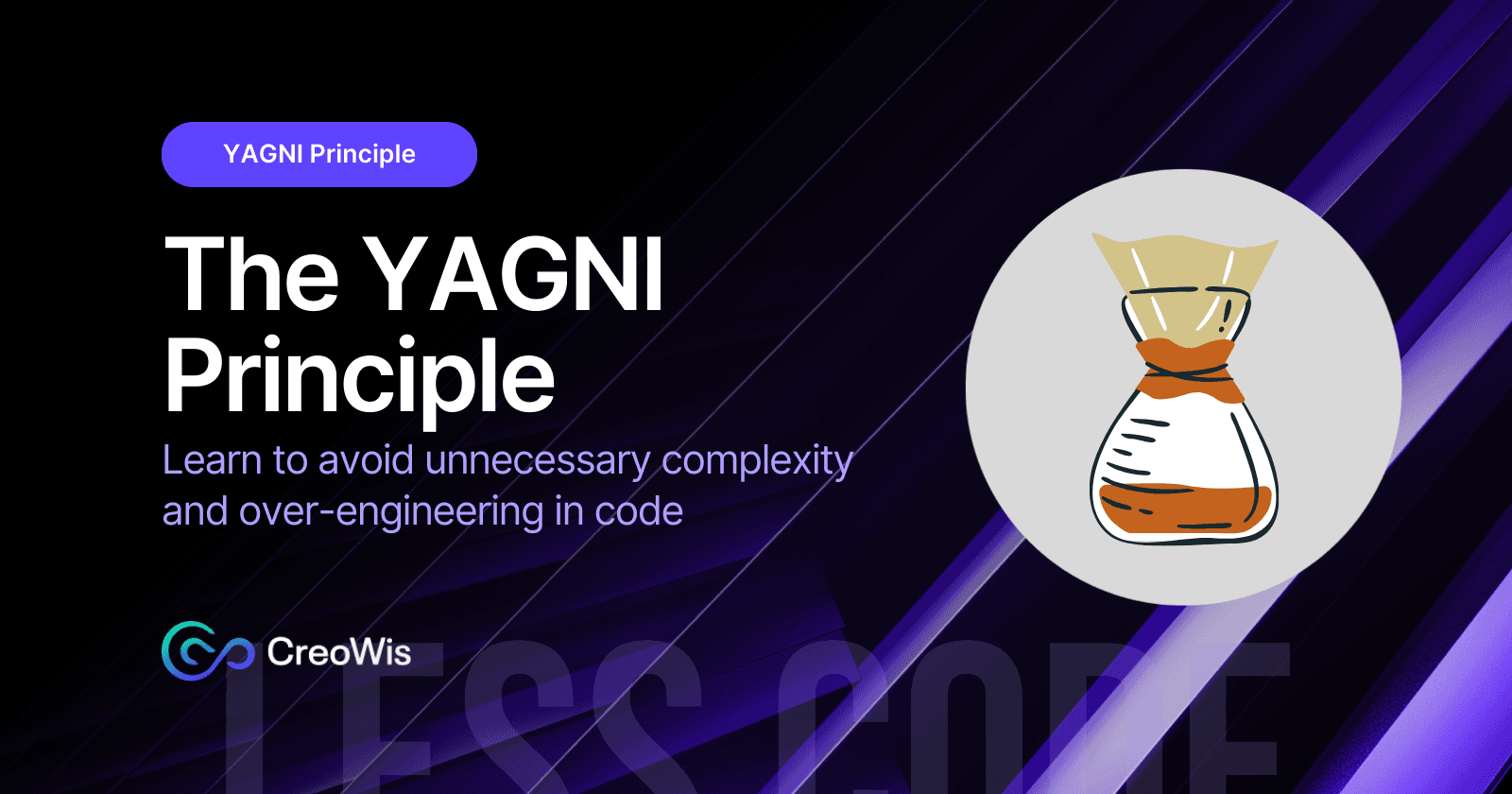
The YAGNI principle in React development
How to avoid unnecessary complexity and over-engineering in code
Introduction
Imagine you’re working on a new feature, and an idea pops into your head: “Maybe I should add this extra function now—it might be useful later.” It seems like a proactive approach, right? After all, future-proofing your code is a good thing, isn’t it?
Not always.
This is where You Ain’t Gonna Need It (YAGNI) comes in. YAGNI is one of the simplest yet most powerful software development principles. It helps developers avoid wasted effort, keeps code simple and maintainable, and reduces complexity and future technical debt.
Yet, many developers, especially ambitious ones, tend to ignore this rule. Why? Because we love solving problems, even ones that don’t exist yet. It’s tempting to build for every possible future scenario, but doing so often leads to bloated, hard-to-maintain code.
What is YAGNI, Really?
Don’t implement something until you actually need it.
However, many misunderstand YAGNI. Some developers think it means never planning ahead, which isn’t true. Others assume it leads to writing lazy, unscalable code, but YAGNI is actually about writing just the right amount of code: no more, no less.
Following YAGNI doesn’t mean ignoring future scalability. It simply ensures that your development process remains focused on solving immediate problems rather than hypothetical ones.
Why Do Developers Over-Engineer?
1. Fear of Future Changes
Developers worry: “What if we need this later?” This fear leads to building unnecessary features that might never be used.
2. Desire to Build the 'Perfect' System
Trying to account for every possible edge case upfront often results in overly complex architectures that slow development down.
3. Lack of Experience
New developers often equate writing more code with writing better code. In reality, simplicity is often more valuable.
Real-World Example
Let's look at some actual code where developers often do too much.
Example 1: The Button That Does Everything
Here's a button component that's trying way too hard:
interface ButtonProps {
label: string;
onClick: () => void;
variant?: 'primary' | 'secondary' | 'tertiary';
size?: 'small' | 'medium' | 'large';
shape?: 'round' | 'square' | 'pill';
elevation?: 'flat' | 'raised' | 'floating';
animation?: 'bounce' | 'pulse' | 'wave';
customClasses?: string;
icon?: React.ReactNode;
iconPosition?: 'left' | 'right';
loading?: boolean;
disabled?: boolean;
tooltipText?: string;
tooltipPosition?: 'top' | 'bottom' | 'left' | 'right';
}
const Button: React.FC<ButtonProps> = ({
label,
onClick,
variant = 'primary',
size = 'medium',
shape = 'square',
elevation = 'flat',
animation,
customClasses,
icon,
iconPosition = 'left',
loading,
disabled,
tooltipText,
tooltipPosition = 'top',
}) => {
return (
<div className="button-wrapper">
{tooltipText && <Tooltip position={tooltipPosition}>{tooltipText}</Tooltip>}
<button
className={`button ${variant} ${size} ${shape} ${elevation} ${animation} ${customClasses}`}
onClick={onClick}
disabled={disabled || loading}
>
{loading && <Spinner />}
{icon && iconPosition === 'left' && icon}
{label}
{icon && iconPosition === 'right' && icon}
</button>
</div>
);
};
Here's what you probably actually need:
interface ButtonProps {
label: string;
onClick: () => void;
variant?: 'primary' | 'secondary';
disabled?: boolean;
}
const Button: React.FC<ButtonProps> = ({
label,
onClick,
variant = 'primary',
disabled = false,
}) => (
<button
className={`button ${variant}`}
onClick={onClick}
disabled={disabled}
>
{label}
</button>
);
Way simpler, right? If you need a loading state later, add it then!
Balancing YAGNI with Future-Proofing
YAGNI doesn’t mean ignoring scalability—it’s about solving real problems, not imaginary ones.
How to Apply YAGNI Without Sacrificing Flexibility:
Keep Code Modular: Design components that can be extended without premature implementation.
Follow Lean Development: Build only what’s necessary and iterate as needed.
Use Feature Toggles: Instead of fully implementing a feature that might not be used, toggle it on when the need arises.
How to Keep It Simple
So, how do you resist the temptation to overengineer? Here are practical strategies:
Ask yourself:
"Do we really need this now?"
"What's the simplest thing that could work?"
Follow the "Rule of Three":
Wait until you need to solve the same problem three times
Then think about making it flexible
Make it work first:
Get it working simply
Then make it better if you need to
Don't try to make it perfect right away
Key Takeaways
Write only what’s needed. Avoid adding features just because they "might be useful."
Refactor when necessary. If new requirements come up, adjust your code when they arrive.
Embrace simplicity. The simplest solution is often the best one.
Following YAGNI helps keep your code clean, maintainable, and efficient—so you can focus on building real solutions instead of solving problems that don’t exist yet!
Final Thoughts: Becoming a YAGNI-First Developer
By embracing YAGNI, you’ll write less code, introduce fewer bugs, and ship faster. Every time you feel tempted to add something just in case, pause and ask yourself: “Are we really gonna need this?”
The best software isn’t the one with the most features; it’s the one that’s easiest to maintain, understand, and extend when the time comes.
Remember:
Simple code = Good code
Add stuff when you need it
Don't guess about the future
Less code = Less bugs
Next time you're coding and think, "maybe we'll need this...", stop and ask "but do we need it right now?"
Chances are, you don't!
Keep it simple and happy coding!
We at CreoWis believe in sharing knowledge publicly to help the developer community grow. Let’s collaborate, ideate, and craft passion to deliver awe-inspiring product experiences to the world.
Let's connect:
This article is crafted by Chhakuli Zingare, a passionate developer at CreoWis. You can reach out to her on X/Twitter, LinkedIn, and follow her work on the GitHub.