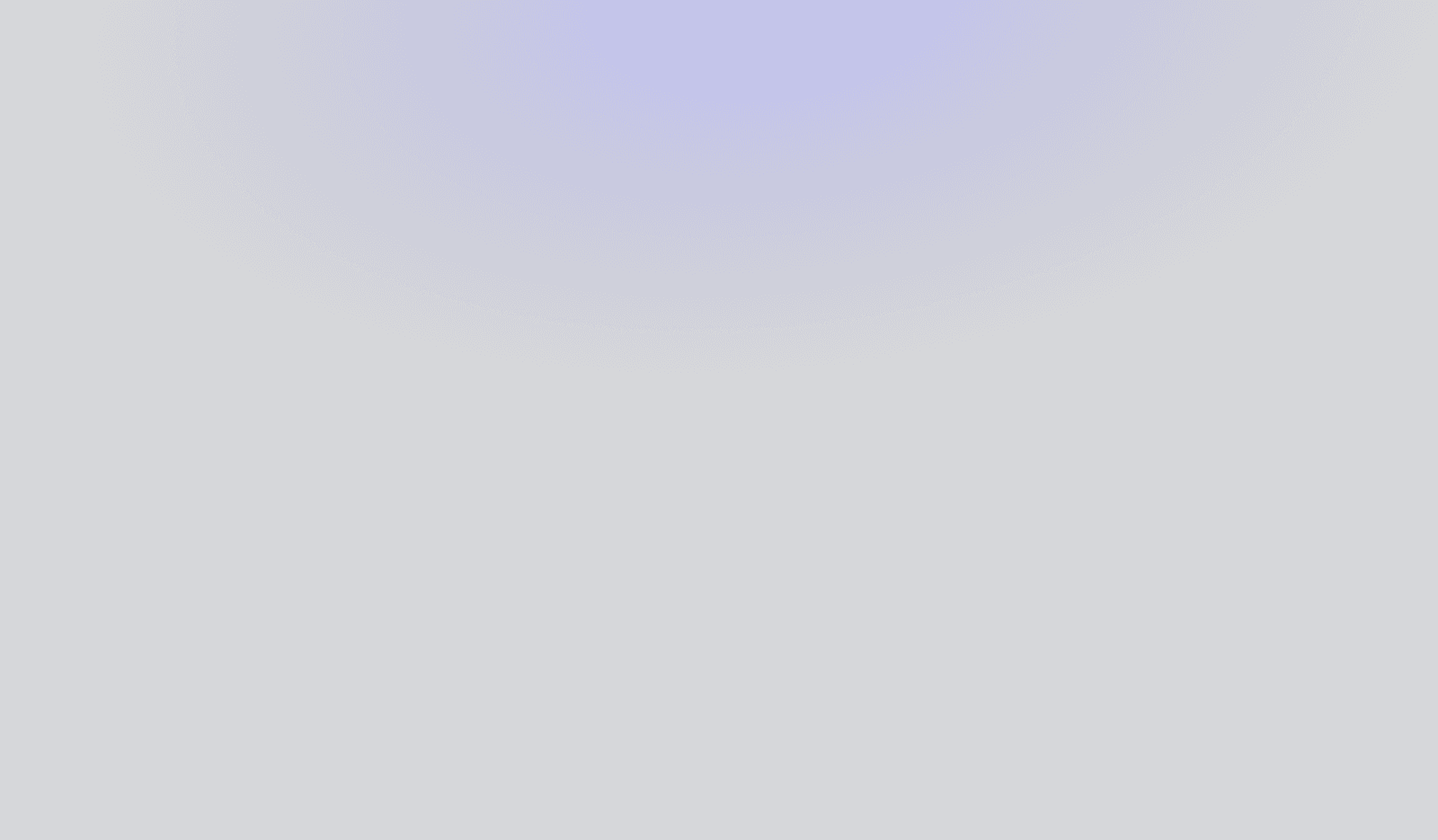
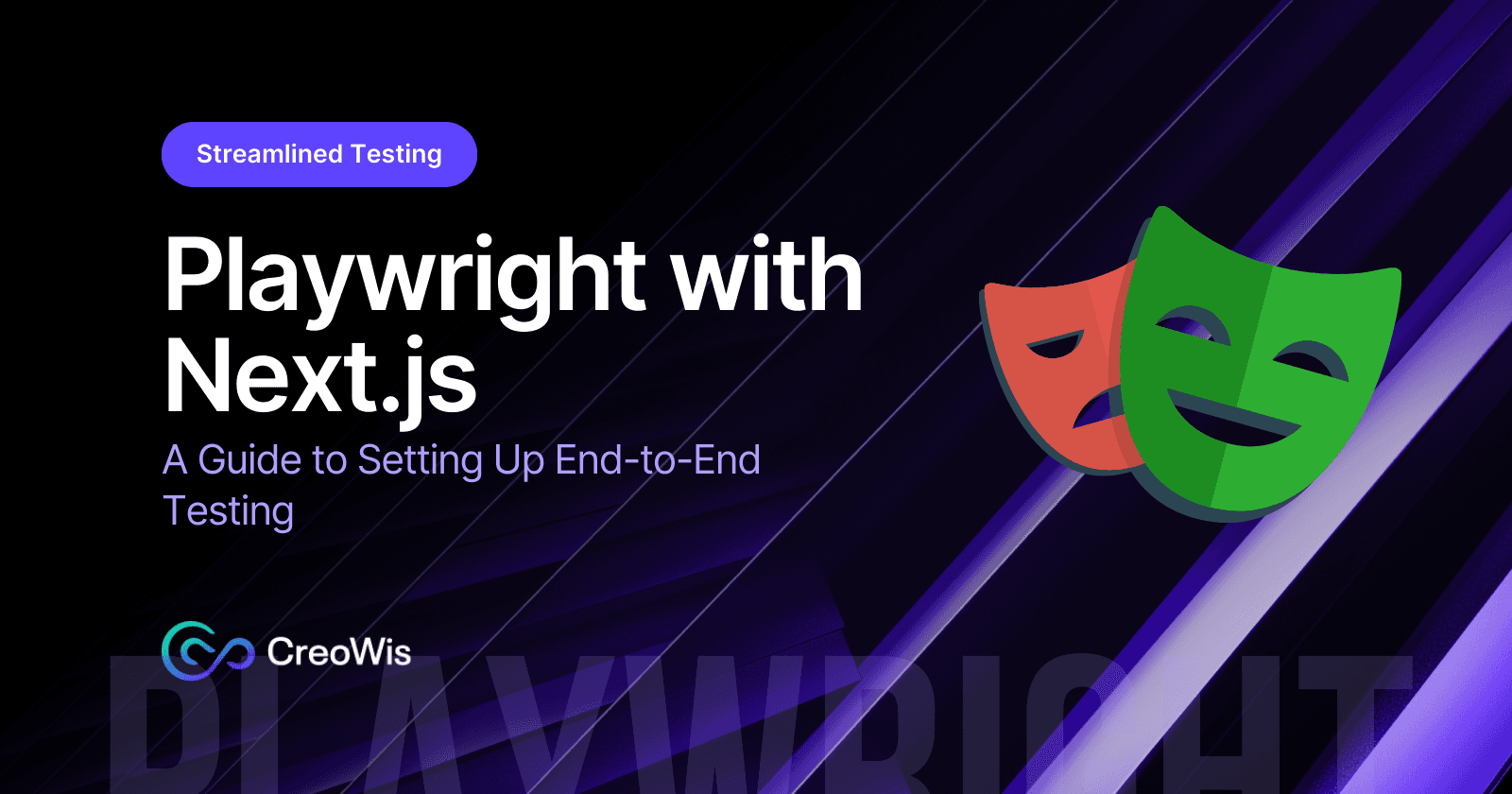
How to Use Playwright with Next.js - A Step-By-Step Guide
A Guide to Setting Up End-to-End Testing in Next.js with Playwright
Greetings from the Playwright universe! You've come to the right spot if you're searching for a robust, dependable, and developer-friendly platform to manage end-to-end (E2E) testing.
Microsoft created Playwright, a cutting-edge testing framework for end-to-end (E2E) testing. It is designed to provide quick, dependable, and adaptable testing for web applications and supports a variety of browsers, including Chrome, Firefox, and WebKit (Safari).
Why Playwright?
For each test, Playwright builds a separate browser context, guaranteeing independence without lag. To increase speed without compromising test isolation, it saves authentication states to avoid repeated logins.
The playwright eliminates the need to use arbitrary timeouts by using web-first assertions and auto-wait for components. To troubleshoot more quickly while testing, set up retries and take screenshots, videos, and traces.
Playwright integrates seamlessly with your tech stack thanks to its support for TypeScript, JavaScript, Python, .NET, and Java. Playwright is a great option for automating user interactions for UI testing.
Setting Up a Next.js Project
We will start by setting up a Next.js project using the Next.js Launchpad of our company, CreoWis. This Launchpad gives you the necessary tools, customizations, and best practices to optimize your development process with a hassle-free, pre-configured setup for Next.js applications. This configuration makes it easier to start a project and lets you concentrate on constructing instead of configuring.
Read more details about what this Launchpad provides here.
Create Project Folder:
Create a new folder for the project, here we will use PlaywrightNext as the project name.
mkdir PlaywrightNext
cd PlaywrightNext
Initialize Next.js with CreoWis Launchpad:
Next, use the CreoWis Launchpad template to set up your Next.js project or you can also use the standard npx create-next-app@latest
command to create a new Next.js project.
npx create-next-app -e https://github.com/CreoWis/next-js-launchpad
Now we are all set to start with Playwright testing to make sure your app runs smoothly, we'll go ahead and use Playwright for end-to-end testing.
Playwright Setup
Let’s get started with installing Playwright, you can do so by using npm, yarn, or pnpm.
npm init playwright@latest
This command will prompt you to:
Choose test directory: This is required. If you press Enter without typing a directory, it will default to tests.
Add GitHub Actions workflow (y/N): Optional—choose "y" if you want automatic CI setup, or press Enter to skip.
Install Playwright browsers (Y/n): Required—press "y" or simply Enter to install the necessary browsers.
Choose each option according to your needs, and the Playwright will complete the setup.
In addition to setting up Playwright, running this command also creates an example test file, example.spec.ts
automatically. A few sample tests that demonstrate basic Playwright features are included in this file, which can be used as a useful guide when you begin creating your tests.
Some Testing Terminologies
Before diving into writing our first Playwright test, let’s understand some basic testing terms and concepts.
Navigation:
The majority of tests start by visiting a URL. This can be done in Playwright by using page.goto()
, which pauses until the page loads before proceeding. You can interact with the page's elements after it has loaded.
await page.goto('https://www.creowis.com');
Interactions:
Locators are used by the Playwright to interact with elements. These enable you to locate and take action on elements, such as filling out a form or clicking a button. Before taking any action, the playwright makes sure the element is ready for interaction.
Some built-in Locators:
page.getByText()
: Locate by text content.page.getByRole()
: Locate by explicit or implicit accessibility attributes.page.getByLabel()
: Locate a form element by the text on its label.page.getByPlaceholder()
: Locate an input by its placeholder.page.getByAltText()
: Locate an element (usually an image) by its alt text.page.getByTitle()
: Locate an element by its title attribute.page.getByTestId()
: Locate an element based on itsdata-testid
attribute.
Actions:
A variety of built-in actions are available in Playwright for interacting with elements. These consist of:
locator.click()
– Click an element.locator.fill()
– Fill an input field.locator.hover()
– Hover over an element.locator.check()
– Check a checkbox.locator.selectOption()
– Select an option from a dropdown.
Assertions:
Conditions in your tests are validated by assertions. For assertions, Playwright offers the expect() function. Some common assertion examples are checking visibility, text existence, or element attributes.
await expect(page.getByText('Elevating ideas with')).toBeVisible();
some common examples of assertions:
expect(locator).toBeChecked()
: Checkbox is checked.expect(locator).toBeVisible()
: Element is visible.expect(locator).toContainText()
: Element contains text.expect(locator).toHaveText()
: Element matches the text.expect(locator).toHaveValue()
: Input element has value.expect(page).toHaveTitle()
: Page has a title.expect(page).toHaveURL()
: Page has URL.
Fixtures:
Fixtures in Playwright are similar to reusable test sets. The environment is automatically set up before the test runs and cleaned up afterwards. To prevent tests from interfering with one another, Playwright, for instance, has an integrated page
fixture that provides you with a new browser page for every test.
test('has title', async ({ page }) => {
await page.goto('https://www.creowis.com/');
const titleText = page.getByText('Elevating ideas with');
await expect(titleText).toBeVisible();
});
Test Hooks:
This is for organizing tests and handling setup/teardown on a broader scale.
Setup: This describes the steps done to get the environment ready before a test run. For instance, starting a browser, going to a particular page, or initializing data.
Teardown: This describes the steps done to tidy up or reset the environment following a test run. This can involve erasing test data or shutting down the browser.
Here are a few of the important ones:
test.describe
: Used to group related tests togethertest.beforeAll
: Runs once before all tests in adescribe
block, typically used for global setup.test.afterAll
: Runs once after all tests in adescribe
block, ideal for global cleanup.test.beforeEach
: Runs before each test, useful for setting up a clean state.test.afterEach
: Runs after each test, ideal for cleanup tasks.
Creating a basic test
Let’s dive right into creating a basic test using Playwright.
test('has subtitle', async ({ page }) => {
await page.goto('https://www.creowis.com/');
const subTitle = page.getByText('Crafting digital experience by');
await expect(subTitle).toBeVisible();
});
Explanation:
test(): Specifies the test case, including an asynchronous function with the test logic and a description ('has subtitle’).
page.goto(): Opens the given URL (in this example, 'https://www.creowis.com/').
page.getByText(): Finds an element by its text that is visible. The subtitle 'Crafting digital experience by' is what it searches for here.
expect(): To establish an assertion and determine whether the element is displayed on the page.
toBeVisible(): An assertion that confirms the element is visible on the page.
The test verifies that the subtitle text appears on the page and is present.
To run the test, use the command:
npx playwright test
If everything is configured properly, the test run should yield a successful result!
It should appear as follows in vs code:
Since it runs on all three browsers for WebKit, Chrome, and Firefox, the count is 3.
The test can also be conducted in UI mode. To do so, execute the command:
npx playwright test --ui
Once your test is finished you also get a comprehensive report of all your tests. If some of the tests fail, the HTML report is automatically viewed by default. To view the report, run the following command:
npx playwright show-report
Bonus:
Try exploring and running the following command to see what Playwright can do:
npx playwright codegen https://www.creowis.com/
Execute this command to perform browser-based actions. Playwright makes it simpler to develop tests by automatically generating code depending on your interactions. Here URL is optional, it is always possible to run the command without the URL and then add the URL straight into the browser window.
Conclusion
With the help of Playwright, developers can easily and effectively automate end-to-end testing. It is an excellent option for testing web applications because of its versatility across many browsers, support for parallel execution, and sophisticated features like network interception.
With this guide, I hope you're ready to start experimenting with Playwright in your projects.
Happy coding!
Resources
We at CreoWis believe in sharing knowledge publicly to help the developer community grow. Let’s collaborate, ideate, and craft passion to deliver awe-inspiring product experiences to the world.
Let's connect:
This article is crafted by Prachi Sahu, a passionate developer at CreoWis. You can reach out to her on X/Twitter, LinkedIn, and follow her work on GitHub.