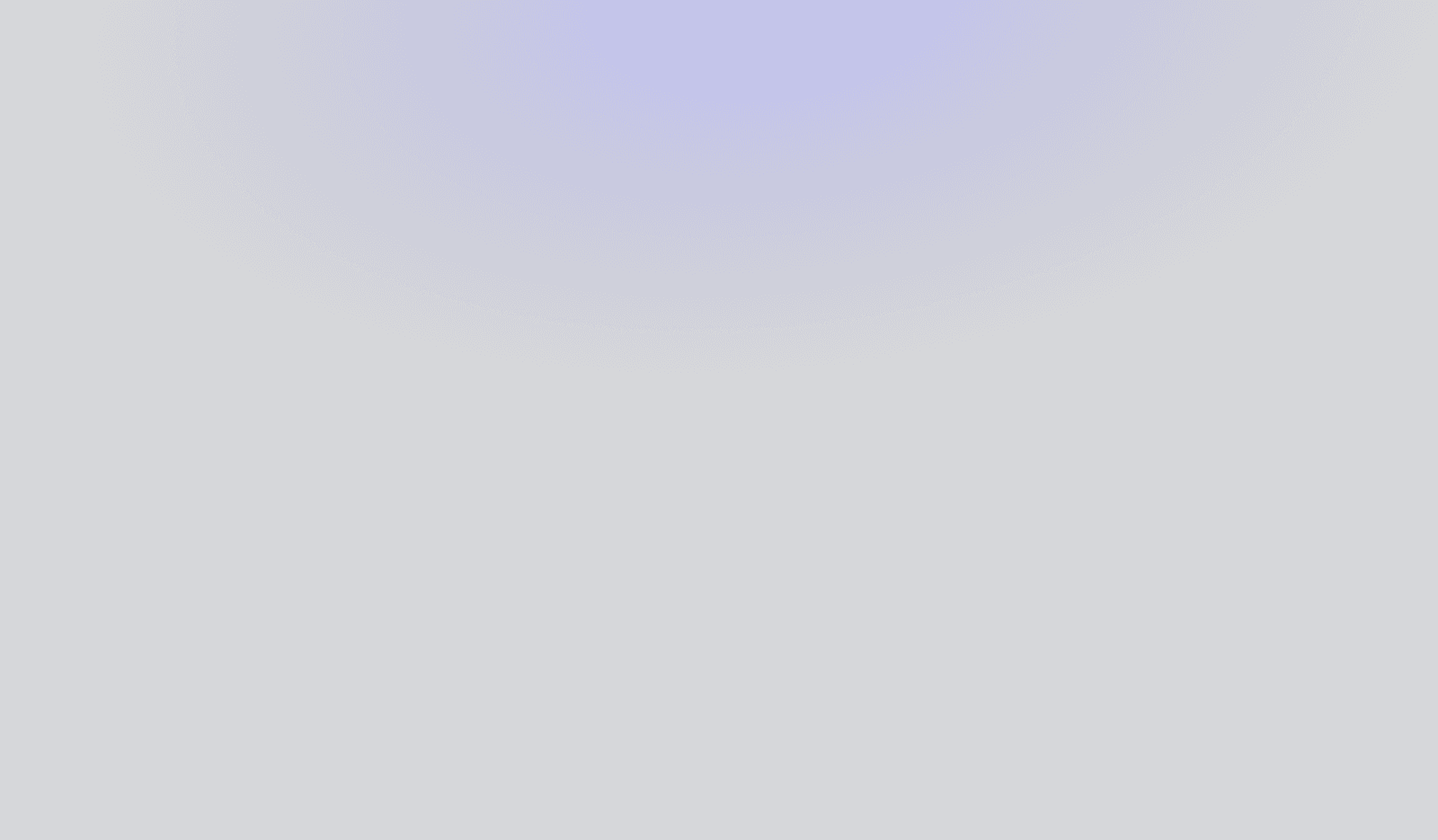
Introduction
API security is crucial for modern web applications, ensuring that sensitive data is protected from unauthorized access, attacks, and misuse. NestJS, a progressive Node.js framework, provides built-in tools and best practices for securing APIs effectively. APIs are vulnerable to threats such as data breaches, unauthorized access, injection attacks, and denial-of-service (DoS) attacks without proper security measures.
In this guide, we’ll explore the key strategies for enhancing API security in NestJS and explain why each measure is essential.
1. Authentication with JWT
Why Use JWT?
Authentication is the first line of defense in securing an API. JSON Web Tokens (JWT) provide a stateless and scalable solution for authentication. Unlike traditional session-based authentication, JWT does not require storing session data on the server, making it an ideal choice for distributed applications. Each token is self-contained and includes user information, expiration time, and a cryptographic signature to prevent tampering.
Implementing JWT in NestJS
Install required packages:
npm install @nestjs/jwt @nestjs/passport passport passport-jwt
Create an AuthModule:
@Module({ imports: [ JwtModule.register({ secret: process.env.JWT_SECRET || 'secretKey', signOptions: { expiresIn: '1h' }, }), ], providers: [AuthService], }) export class AuthModule {}
Implement JWT strategy:
@Injectable() export class JwtStrategy extends PassportStrategy(Strategy) { constructor(private readonly authService: AuthService) { super({ jwtFromRequest: ExtractJwt.fromAuthHeaderAsBearerToken(), secretOrKey: process.env.JWT_SECRET || 'secretKey', }); } async validate(payload: any) { return { userId: payload.sub, username: payload.username }; } }
Implementing JWT authentication ensures that only users with valid tokens can access protected resources. This prevents unauthorized access and strengthens overall API security.
2. Role-Based Access Control (RBAC)
Why is RBAC Important?
In any application, not all users should have the same level of access to resources or functionality. For example, an admin might need access to sensitive data or administrative actions, while a regular user should only have access to their own data. Role-Based Access Control (RBAC) is a security mechanism that enforces permissions based on user roles. It ensures that only authorized individuals can perform specific actions.
Without RBAC, attackers could potentially exploit vulnerabilities to gain unauthorized access to sensitive data or perform actions they shouldn’t be allowed to. For instance, a regular user might gain admin privileges and manipulate or delete critical data. RBAC helps prevent such scenarios by restricting access based on predefined roles.
1. Define User Roles
First, you need to define the roles that users can have in your application. This is typically done using an enum
in TypeScript.
export enum UserRole {
Admin = 'admin', // Administrator role
User = 'user', // Regular user role
}
Here, we’ve defined two roles:
Admin: Users with administrative privileges.
User: Regular users with limited access.
2. Create a Role Guard
A Guard in NestJS is a class that determines whether the route handler should handle a request or not. You can create a custom guard to enforce role-based access control.
Here’s how you can create a RolesGuard
:
import { Injectable, CanActivate, ExecutionContext } from '@nestjs/common';
import { Observable } from 'rxjs';
import { UserRole } from './user-role.enum'; // Import the UserRole enum
@Injectable()
export class RolesGuard implements CanActivate {
canActivate(context: ExecutionContext): boolean {
const request = context.switchToHttp().getRequest();
const user = request.user; // Assuming the user object is attached to the request
// Check if the user has the 'admin' role
return user?.role === UserRole.Admin;
}
}
How It Works:
The
canActivate
method is called whenever a request is made to a route protected by this guard.It retrieves the
user
object from the request (this is typically added by an authentication middleware or guard).It checks if the user’s role matches the required role (
Admin
in this case).If the user has the required role, the request is allowed to proceed. Otherwise, it is denied.
3. Apply the Guard to Routes
Once the guard is created, you can apply it to specific routes or controllers to restrict access based on roles.
For example, let’s protect an admin-only endpoint:
import { Controller, Get, UseGuards } from '@nestjs/common';
import { RolesGuard } from './roles.guard';
@Controller('admin')
@UseGuards(RolesGuard) // Apply the RolesGuard to all routes in this controller
export class AdminController {
@Get('data')
getAdminData() {
return 'This is sensitive admin data.';
}
}
What Happens Here:
The
@UseGuards(RolesGuard)
decorator ensures that theRolesGuard
is applied to all routes in theAdminController
.When a request is made to the
/admin/data
endpoint, theRolesGuard
checks if the user has theAdmin
role.If the user is an admin, they can access the data. Otherwise, they will receive a
403 Forbidden
error.
4. Adding Flexibility for Multiple Roles
The above example only checks for the Admin
role. If you want to support multiple roles, you can make the guard more flexible. For example:
import { Injectable, CanActivate, ExecutionContext } from '@nestjs/common';
import { Reflector } from '@nestjs/core';
import { UserRole } from './user-role.enum';
@Injectable()
export class RolesGuard implements CanActivate {
constructor(private reflector: Reflector) {}
canActivate(context: ExecutionContext): boolean {
const requiredRoles = this.reflector.get<UserRole[]>('roles', context.getHandler());
if (!requiredRoles) {
return true; // No roles required, allow access
}
const request = context.switchToHttp().getRequest();
const user = request.user;
return requiredRoles.includes(user?.role); // Check if the user has one of the required roles
}
}
How It Works:
The
Reflector
is used to retrieve metadata (in this case, the required roles) attached to the route handler.You can specify the required roles using a custom decorator (e.g.,
@Roles(UserRole.Admin, UserRole.Editor)
).The guard checks if the user’s role matches any of the required roles.
5. Custom Decorator for Roles
To make it easier to specify roles for routes, you can create a custom decorator:
import { SetMetadata } from '@nestjs/common';
import { UserRole } from './user-role.enum';
export const Roles = (...roles: UserRole[]) => SetMetadata('roles', roles);
Now you can use this decorator to specify roles for specific routes:
@Controller('admin')
export class AdminController {
@Get('data')
@Roles(UserRole.Admin) // Only admins can access this route
getAdminData() {
return 'This is sensitive admin data.';
}
}
Key Benefits of RBAC in NestJS
Granular Access Control: You can define specific roles and permissions for different parts of your application.
Improved Security: Prevents unauthorized users from accessing sensitive data or performing restricted actions.
Scalability: As your application grows, you can easily add new roles and permissions without rewriting your access control logic.
3. Input Validation & Data Sanitization
Why is Input Validation Important?
Unchecked user input is one of the most common attack vectors for web applications. Attackers exploit vulnerabilities like SQL Injection, Cross-Site Scripting (XSS), and Command Injection by injecting malicious input into API endpoints. Proper validation ensures that user-provided data adheres to expected formats and prevents security loopholes.
Using Validation in NestJS
Install class-validator:
npm install class-validator class-transformer
Define DTO with validation rules:
export class CreateUserDto { @IsString() @Length(3, 20) username: string; @IsEmail() email: string; @IsString() @MinLength(6) password: string; }
Apply validation in controllers:
@Post('register') createUser(@Body(new ValidationPipe()) createUserDto: CreateUserDto) { return this.authService.createUser(createUserDto); }
This helps prevent malformed or malicious data from being processed, protecting your API from common security threats.
4. Securing API Endpoints with Rate Limiting
Why is Rate Limiting Essential?
APIs can be overwhelmed by excessive requests, leading to service disruptions and denial-of-service (DoS) attacks. Rate limiting controls the number of requests an API accepts within a given timeframe.
Implementing Rate Limiting in NestJS
Install rate-limiting middleware:
npm install express-rate-limit
Configure rate limiting:
import * as rateLimit from 'express-rate-limit'; app.use(rateLimit({ windowMs: 15 * 60 * 1000, // 15 minutes max: 100, // Limit each IP to 100 requests per window }));
This protects against brute-force attacks and ensures that your API remains available to legitimate users.
Conclusion
Securing your NestJS API is a continuous process that involves multiple layers of protection. By implementing JWT authentication, role-based access control, input validation, CSRF protection, rate limiting, HTTPS enforcement, and proper logging, you can ensure a secure and reliable API.
Security breaches can lead to data leaks, financial losses, and reputational damage. Implementing these best practices safeguards user data and maintains system integrity. Always monitor and update your security mechanisms as threats continue to evolve.