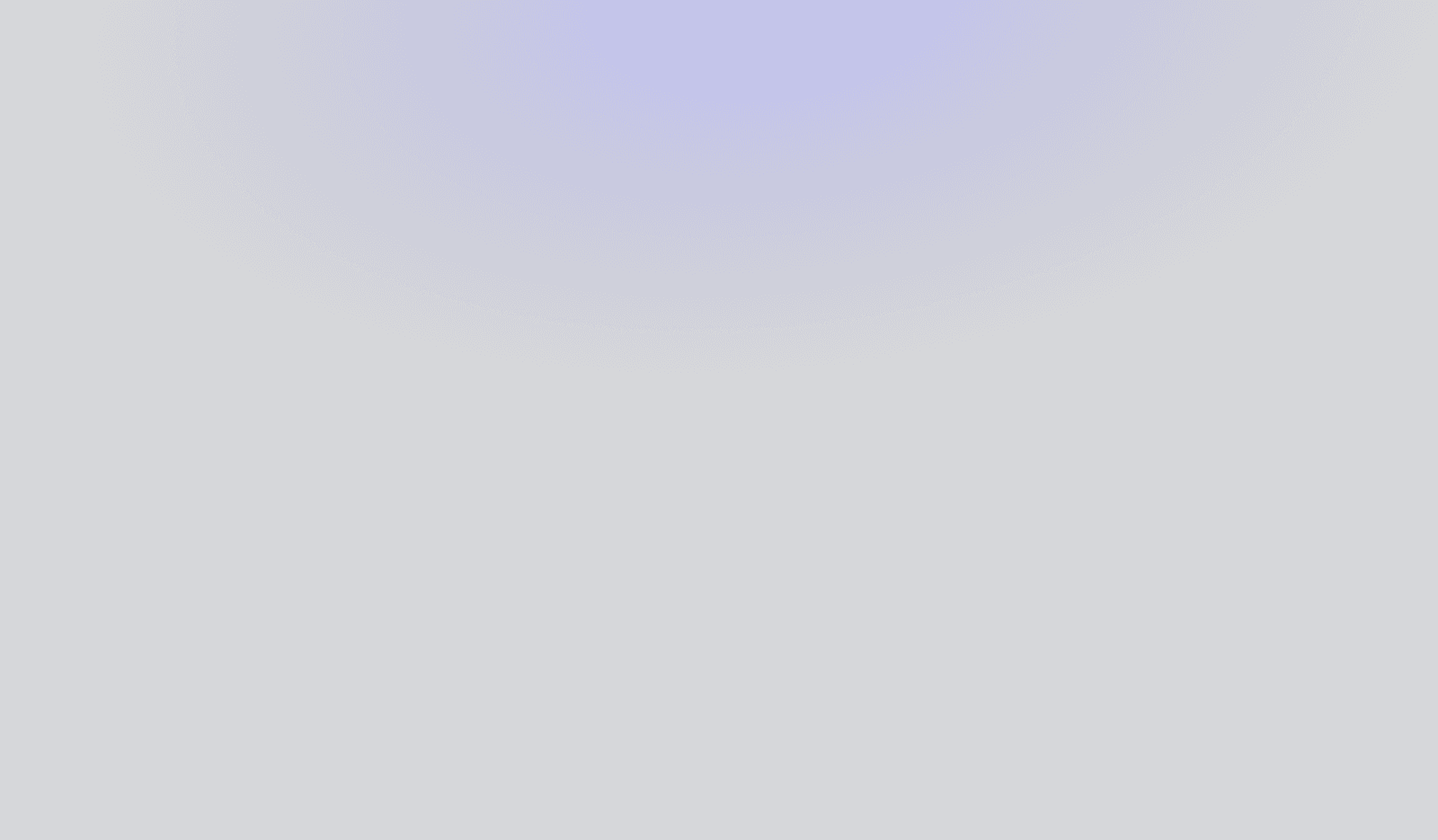
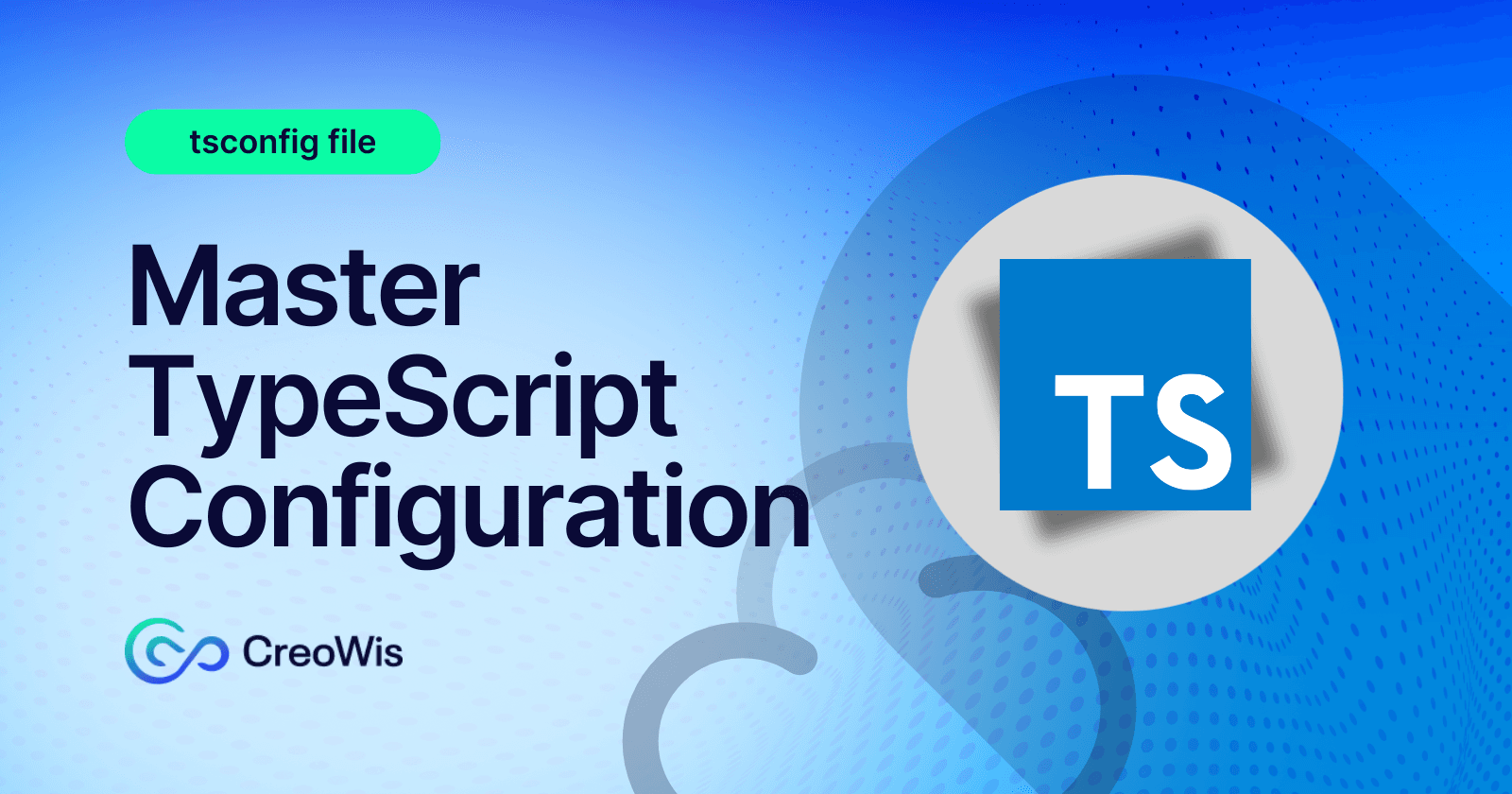
Deep dive into the TypeScript Configurations(tsconfig file)
Understanding the Core of TypeScript Configurations (tsconfig file)
In today's digital era, every business needs an online presence through a website and applications. A website can go live instantly, allowing businesses to make a soft or hard launch in the market.
In fact, Amazon launched its business through a website on July 16, 1995. Initially, it was an online marketplace for books, and the first book sold on Amazon.com was Douglas Hofstadter's Fluid Concepts and Creative Analogies.
Later, Amazon expanded by developing mobile applications for Android and iOS, showcasing how businesses grow with the help of a website. This is why most businesses launch their services or products on a website first.
To build a website, we need HTML, CSS, and JavaScript, the foundational technologies of the web. Using these three, we can create almost any website. Of course, here we are referring specifically to frontend development.
JavaScript dominates the web when it comes to building dynamic and interactive websites. If we call JavaScript the heart of the web, we wouldn’t be wrong. I once came across a quote on the internet that perfectly sums it up:
JavaScript is the duct tape of the internet!
However, JavaScript is not limited to frontend development only. It has been widely adopted across multiple domains. A few years ago, I shared a post on Twitter about JavaScript's extensive usage in different fields, including web development, app development, and more.
The Problem with JavaScript
JavaScript has several issues, but since this blog focuses on the tsconfig.json
file, we will specifically discuss one of its most unexpected behaviors, which is being dynamically typed (loosely typed).
let num = 99;
num = "Hello world"; // No error in JavaScript
In the above code, JavaScript doesn’t throw an error. Initially, num
holds a number, but later, it stores a string without any warning.
This lack of strict type rules can cause unexpected bugs when variables are used differently than planned.
Many tools and technologies are available to address JavaScript's limitations. However, to specifically solve the dynamic typing problem, Microsoft introduced TypeScript, a technology designed to bring static typing and better tooling to JavaScript.
Why TypeScript?
TypeScript is not a standalone language; instead, it is a superset of JavaScript. This means it includes all JavaScript features while introducing additional capabilities to improve development.
In the end, all TypeScript code is compiled into plain JavaScript, making it executable in any JavaScript environment.
TypeScript acts as a powerful “tool” for developers by offering:
Early Bug Detection – Catches type-related errors at compile time, reducing runtime issues.
Improved Code Readability – With explicit types, the code becomes easier to understand and maintain.
Better Scalability for Large Projects – Helps structure and manage complex codebases efficiently.
Enhanced Developer Experience – Provides auto-completion, IntelliSense, and better navigation in modern IDEs, such as VS Code.
Modern JavaScript Features – Supports ES6+ features before some browsers fully implement them.
Object-Oriented Programming (OOP) Support – Features like interfaces improve code organization.
With these advantages, TypeScript helps developers write cleaner, more reliable, and maintainable code, making it the preferred choice for many modern projects.
Installing TypeScript
To start using TypeScript, we need to install it first. There are multiple ways to install TypeScript depending on your setup.
However, the recommended way to install TypeScript is via NPM. If you have Node.js installed, NPM comes bundled with it. Run the following command in your terminal:
npm install -g typescript
Here, The -g
flag installs TypeScript globally, allowing you to use it in any project.
After installation, check if TypeScript is installed correctly by running the below command:
tsc --v
If TypeScript is installed, this will display the installed version in your terminal. Now TypeScript is successfully installed, we are ready to move ahead! 🚀
Different Ways to Compile TypeScript
Once TypeScript is installed, we need to compile our .ts
files into JavaScript (.js
). TypeScript provides multiple ways to do this based on your workflow and project size.
- Compiling a Single File: The simplest way to compile a TypeScript file is:
tsc index.ts
This generates a corresponding JavaScript file index.js
in the same directory. However, if we modify index.ts
, we must manually re-run the command to update index.js
.
- Compiling Multiple Files: Instead of compiling files one by one, we can compile multiple files at once by specifying them with spaces:
tsc index.ts app.ts util.ts
This command compiles index.ts
, app.ts
, and util.ts
into their respective JavaScript files. But doing this every time is tedious, right?
- Watching a Single File for Changes (
--watch
or-w
flag): Instead of manually compiling every time, we can use watch mode, which automatically re-compiles whenever the file is modified:
tsc index.ts --watch
or simply:
tsc index.ts -w
- Watching Multiple Files for Changes: We can also enable watch mode for multiple files:
tsc index.ts app.ts util.ts -w
Now, whenever we modify index.ts
, app.ts
, or util.ts
, TypeScript will automatically recompile them into JavaScript.
Challenges with Manual Compilation
While these commands work for small projects, but they have limitations:
Inefficient for large projects: Manually compiling files one by one is time-consuming.
Forgetting to recompile: If we modify
index.ts
, we must re-runtsc index.ts
to updateindex.js
. Forgetting this step can lead to outdated JavaScript, causing unexpected behavior.Lack of custom configurations: We can’t control settings like module resolution, output directory, or strict type checking.
Solution?
To overcome these challenges, we use tsconfig.json
file, which allows us to configure and automate TypeScript compilation efficiently.
What is tsconfig.json
file
TypeScript provides a powerful way to write scalable and maintainable code, but managing multiple files and configurations manually can become complex. This is where tsconfig.json
comes in!
A tsconfig.json
file is a JSON configuration file that defines the compiler options and root files for a TypeScript project. It acts as a centralized way to specify how TypeScript should compile the code.
Without tsconfig.json
, developers must manually pass compiler options every time they run tsc
, which is repetitive and error-prone.
Additionally, TypeScript projects often require:
Automated Compilation – Instead of manually compiling each file using
tsc index.ts
, we can simply runtsc
to compile the entire project based on predefined settings intsconfig.json
file.Consistent Configuration – Ensures that all developers on a team use the same TypeScript compiler settings, reducing inconsistencies across environments.
Strict Type Checking – Enforces stricter rules catching potential errors at compile time rather than runtime.
Better Project Structure – Allows specifying which files should be compiled, where the output should be stored, and how modules should be resolved.
Improved Performance – Enables incremental builds, which means TypeScript only recompiles changed files instead of reprocessing the entire project, improving speed.
Watch Mode (
tsc -w
) – Instead of runningtsc
manually after every change, enabling watch mode allows TypeScript to automatically recompile files whenever they are modified.Module & Target Control – Allows configuring which module system and JavaScript version TypeScript should compile to, ensuring compatibility with different runtimes.
The tsconfig.json
file should be placed at the root of the project, especially for projects with multiple TypeScript files.
When we run:
tsc
TypeScript automatically looks for tsconfig.json
and applies its configurations instead of requiring manual command-line options.
How to create tsconfig.json
file
Now we understand the need for tsconfig.json
, let’s create one. Run the following command in the root directory of your project:
tsc --init
This will generate a tsconfig.json
file with default configurations. We can now modify it as per your project requirements.
Key Properties in tsconfig.json
file
The tsconfig.json
file consists of various properties that define how TypeScript compiles the project.
Below are some of the most essential properties:
compilerOptions
The compilerOptions
property defines various settings that determine how TypeScript should compile the code:
{
"compilerOptions": {
// Define various settings as JSON properties based on the project requirements
}
}
In the above configuration, the compilerOptions
section allows us to define numerous properties that control different aspects of the TypeScript compilation process.
Based on these properties, the TypeScript compiler compiles the code.
files
The files
property specifies individual TypeScript files to be compiled. If this property is used, only the listed files will be compiled.
{
"files": [
"index.ts",
"app.ts"
]
}
In the above configuration, only index.ts
and app.ts
will be compiled by the TypeScript compiler. If any of the specified files are missing from the directory, an error will be thrown.
extends
The extends
property allows a tsconfig.json
file to inherit settings from another configuration file. This helps in maintaining a consistent setup across multiple projects while avoiding redundant configurations.
The base file is loaded first, and then the inheriting file overrides specific settings. However, properties like files
, include
, and exclude
in the inheriting file completely replace those from the base file.
Base configuration (configs/base.json
): This file contains common settings that multiple projects can reuse.
{
"compilerOptions": {
// Base settings for all projects
}
}
This acts as the foundation, and other configurations can extend it.
Project-specific configuration (tsconfig.json
): A project-specific file that extends the base config and adds its own file list.
{
"extends": "./configs/base",
"files": ["main.ts", "index.ts"]
}
This file inherits all settings from configs/base.json
but defines which files should be compiled for this particular project.
"extends": "./configs/base"
: This instructs TypeScript to load the settings frombase.json
first."files": ["main.ts", "index.ts"]
: Instead of compiling all.ts
files in the directory, onlymain.ts
andindex.ts
will be compiled by the TypeScript compiler. Any other files in the directory will be ignored unless they are explicitly listed.include
&exclude
The include and exclude properties in
tsconfig.json
allow us to specify exactly which files the TypeScript compiler should process. This helps make the build efficient by including only the needed files and leaving out the rest.include
: Theinclude
property is a list of patterns that tells TypeScript which files or folders to compile.exclude
: Theexclude
property is a list of patterns that tells TypeScript which files or folders to skip during compilation.
{
"include": ["src/**/*"],
"exclude": ["node_modules", "dist"]
}
"include": ["src/**/*"]
: This tells TypeScript to compile every file that is located in thesrc
folder and any of its subdirectories."exclude": ["node_modules", "dist"]
: This instructs the compiler to ignore files in thenode_modules
folder and thedist
folder.references
The
references
property helps organize large projects by connecting multiple TypeScript projects.It lets you split a big codebase into smaller, manageable parts, which improves build times and organization.
Each linked project must have
"composite": true
in itstsconfig.json
file, so TypeScript can create the needed build information.
{
"compilerOptions": {
"composite": true
},
"references": [
{ "path": "./project-1" },
{ "path": "./project-2" }
]
}
In this setup, TypeScript will compile the projects in project-1
and project-2
first, before compiling the main project. This makes sure all type declarations and build outputs are current. This approach simplifies the build process and keeps the project organized.
For more information, you can look at the official documentation on project references.
Configuring Compiler Options
TypeScript becomes a more powerful tool when you manually configure it according to your project's requirements. You can set various rules in the compilerOptions
section of your tsconfig.json
file. These settings control how TypeScript compiles your code and determine the output JavaScript.
Below, I will share one of the key configurations you should know. Remember, this list isn't complete, so always check the official documentation for more options and details.
target
:
The target
compiler option specifies the version of JavaScript that the TypeScript compiler will output. For example, setting "target": "ES3"
instructs the compiler to produce ES3-compatible JavaScript.
{
"compilerOptions": {
"target": "ES3" // Options: ES3, ES5, ES6, ESNext, etc.
}
}
You can also set the target
to other versions. For instance, ESNext
refers to the highest JavaScript version your current TypeScript version supports.
When the target
is set to an older version like ES3, features like const
, let
, and arrow functions aren't supported by default. But the TypeScript compiler will automatically convert modern JavaScript features into code that works with the specified target.
For example, consider the following modern JavaScript code written in TypeScript:
const a = 534;
const sum = () => {
return 5 + 10;
};
If you compile this code with "target": "ES3"
, it might be transformed into:
"use strict";
var a = 534;
var sum = function () {
return 5 + 10;
};
Here, the const
declaration is converted into var
, and the arrow function is transformed into a standard function expression. This ensures that your code runs on older browsers that do not support ES6 features.
removeComments
:
The removeComments
option removes comments from the compiled JavaScript output.
{
"compilerOptions": {
"removeComments": true,
}
}
The "removeComments": true
setting instructs TSC to remove all comments from the compiled JavaScript files.
As shown above, TSC removes all comments from the JavaScript output.
noUnusedParameters
:
The noUnusedParameters
option reports errors for any unused function parameters.
{
"compilerOptions": {
"noUnusedParameters": true,
}
}
The "noUnusedParameters": true
setting instructs TSC to flag any function parameters that are not used within the function body.
For example, if a function has three parameters (a
, b
, and c
), and one or more are unused, you'll see a squiggly line under the unused parameters.
noUnusedLocals
:
The noUnusedLocals
option reports errors for unused local variables.
{
"compilerOptions": {
"noUnusedLocals": true,
}
}
The "noUnusedLocals": true
setting instructs TSC to flag any local variables that are declared but not used.
Similar to noUnusedParameters
, the noUnusedLocals
option will show a warning with a squiggly line. if there are local variables that are declared but not used.
outDir
:
The outDir
option specifies the directory where the compiled JavaScript files will be saved.
{
"compilerOptions": {
"outDir": "./dist",
}
}
The "outDir": "./dist"
setting instructs the TypeScript compiler to place all the compiled JavaScript files into the dist
folder.
For example, if TSC compiles /src/index.ts
, the resulting file will be saved as /dist/index.js
.
rootDir
:
The rootDir
option specifies the root directory of your TypeScript source files.
{
"compilerOptions": {
"rootDir": "utils"
}
}
In this setup, "rootDir": "utils"
tells TSC to use the utils
folder as the main directory for TypeScript files. This means only files inside the utils
folder will be compiled, and their folder structure will stay the same in the output.
As a result, when you use an outDir
setting like "outDir": "./dist"
, TSC will compile the files from the utils
folder and put the JavaScript files into the dist
folder, keeping the folder structure that starts from utils
.
This list of configuration options does not end here; depending on your project's requirements and structure, you can further customize TypeScript settings to suit your needs.
Summary
In this blog, we looked at how TypeScript improves JavaScript development by fixing dynamic typing problems and providing strong features like early bug detection and better code organization. We discussed how to install TypeScript and different ways to compile your TypeScript files, from compiling a single file to using watch mode for automatic recompilation.
We also explored the tsconfig.json
file, which is a main configuration file that makes managing TypeScript projects easier. We covered important properties like compilerOptions
, files
, extends
, include
, exclude
, and references
. We also talked about specific compiler options such as target
, removeComments
, noUnusedParameters
, noUnusedLocals
, outDir
, and rootDir
. These settings allow you to customize how your code is compiled, organize your project, and keep your code consistent and efficient.
While this guide covers many important settings, there's always more to learn. For more options and detailed information, make sure to check the official TypeScript documentation.
We at CreoWis believe in sharing knowledge publicly to help the developer community grow. Let’s collaborate, ideate, and craft passion to deliver awe-inspiring product experiences to the world.
Let's connect:
This article is crafted by Ajay Yadav, a passionate developer at CreoWis. You can reach out to him on X/Twitter, LinkedIn, and follow him work on the GitHub.