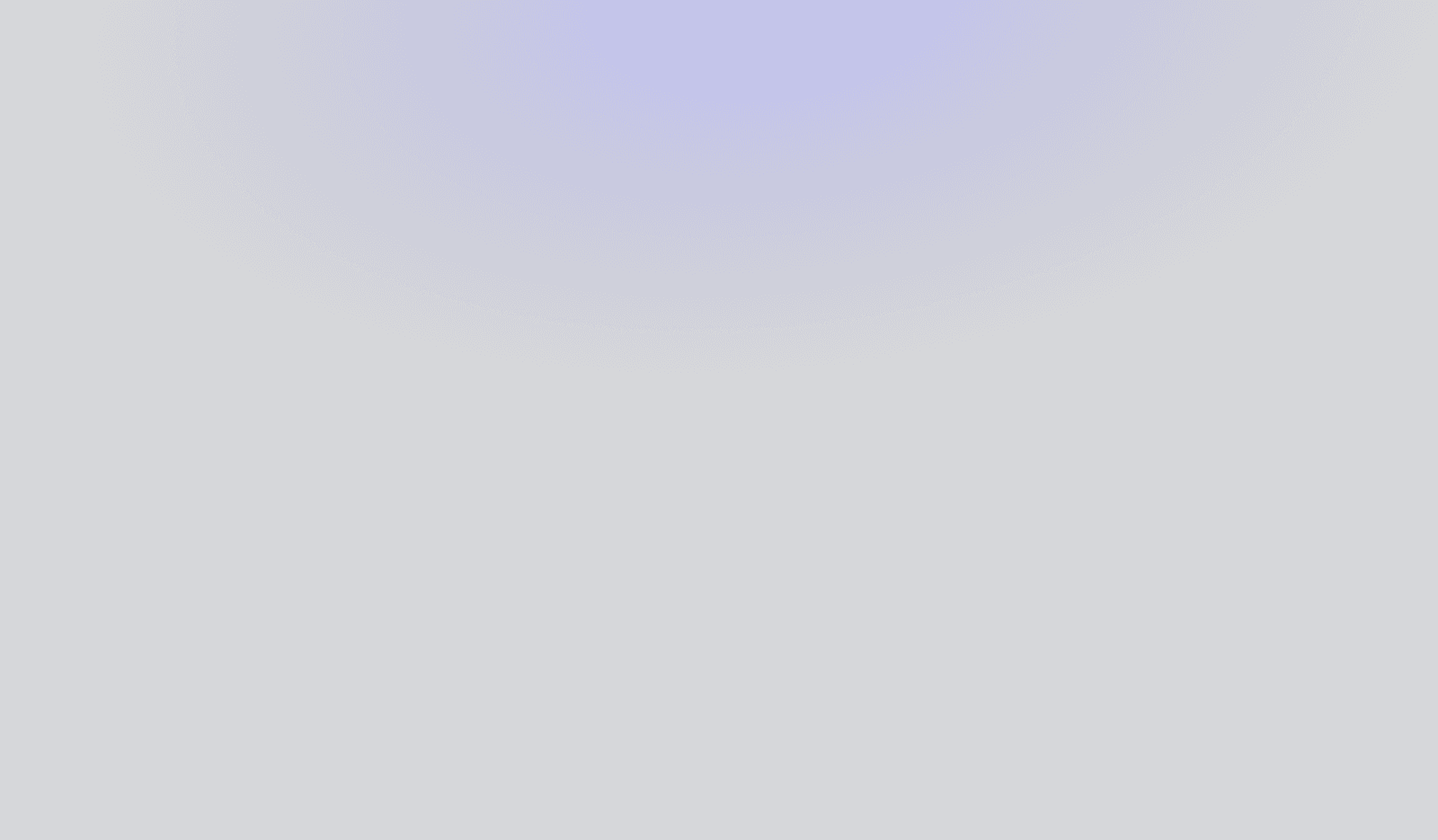
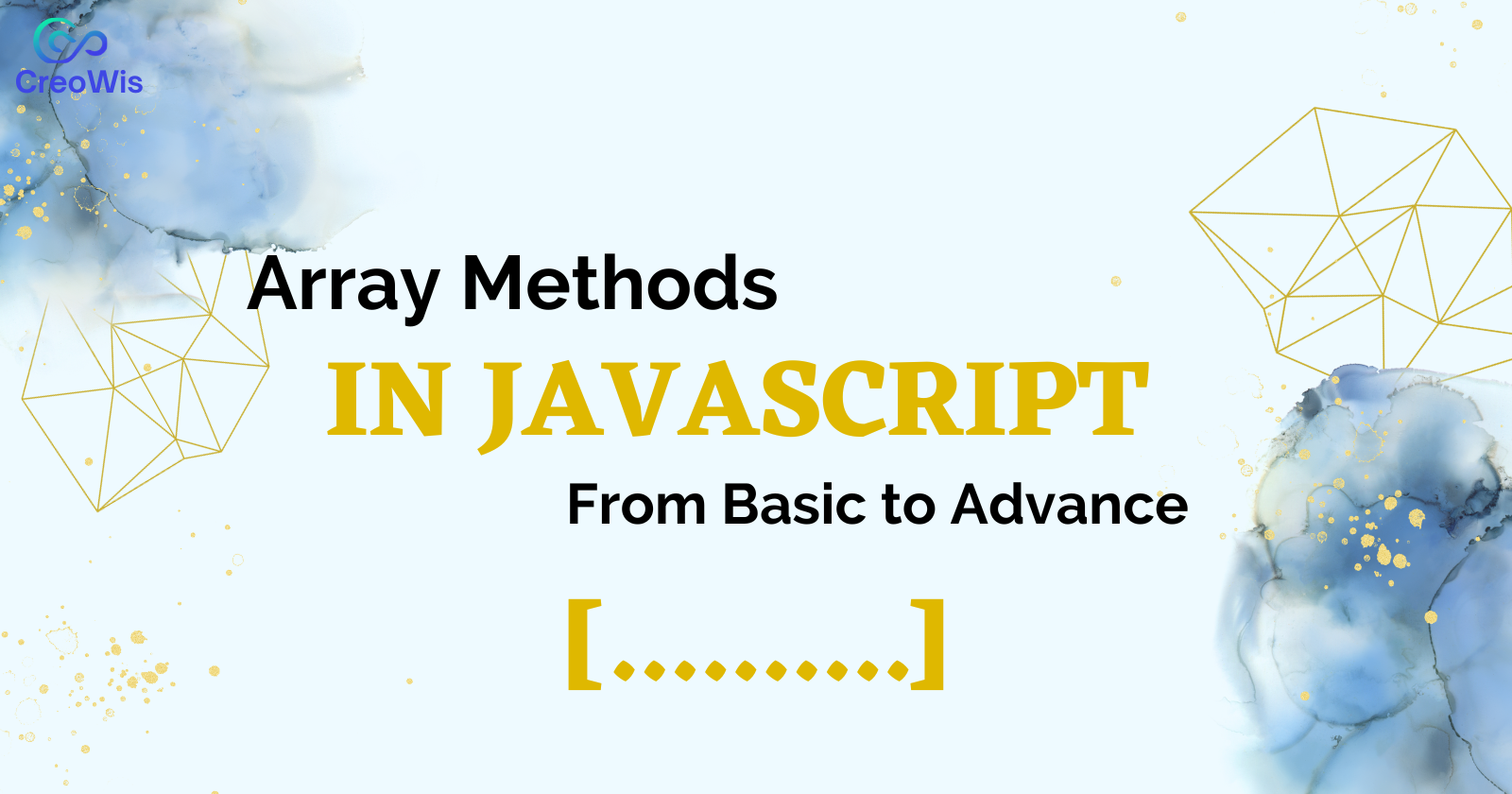
A comprehensive guide to JavaScript array methods
Learn about the JavaScript array methods with example and explanations. You will learn basic to advanced usages of these methods.
Introduction to JavaScript Arrays
Welcome to the thrilling world of JavaScript programming! If you're here, you're obviously curious about JavaScript arrays and the many techniques you can use to manipulate them. And trust me, they are as cool as they sound!
Here, we will be journeying through the fundamentals all the way through to the advanced stuff. So let's put on our learning hats and dig right in! 🎩💻
JavaScript array methods – a powerful set of tools that can take your coding game to the next level! Whether you're a seasoned developer or just starting out, understanding and utilizing these array methods is essential for creating efficient, clean, and dynamic code
What are JavaScript Arrays?
First things first, what exactly are JavaScript arrays?
Put simply, an array is a single variable that is used to store different elements. It has a lot of components but the key ones are:
It’s an ordered list of values.
Each value (also known as an element) has a numeric position, known as its index.
Arrays can store elements of any type, including numbers, strings, and objects.
Think of an array as a big box with many compartments, where you can neatly store anything you want, and each has a specific number (index) so you can find it easily later.
Basic JavaScript Array methods
JavaScript is a treasure box full of useful tools, and array methods are some of its most valuable gems💎. When working with JavaScript arrays, you get an array of use cases to consider. Let’s dive into the basic JavaScript array methods, which will not only be handy but can enhance your efficiency and clean up your code.
The forEach() method - the navigator
The first method worth mentioning is the forEach method. It is essentially a loop, running the same function on each item in an array, one by one. It’s simple, effective, and makes iterating through an array a breeze.
A simple example would look like this:
const array = [1, 2, 3, 4, 5];
array.forEach(item => console.log(item));
This code will print, number by number, every item of the array to the console.
The map() method - the transformer
The map method takes an array, performs a function on each item, and then returns a new array with the results. Imagine having a list of raw data and turning it into useful information, all with a single line of code.
Here’s how it works:
const words = ['hello', 'world'];
const capitalizedWords = words.map(word => word.toUpperCase());
console.log(capitalizedWords);
// Result: ['HELLO', 'WORLD']
We've just capitalized each item in our list and created a fresh new array with the results!
The filter() method - the gatekeeper
With JavaScript's filter method, you can easily sift an array based on a specific condition, returning a new array with the items that match the criteria.
Below is an example:
const array = [1, 2, 3, 4, 5];
const newArray = array.filter(item => item <= 3);
console.log(newArray );
// Output: [1, 2, 3]
The reduce() method - the accumulator
The reduce method in JavaScript turns an array into a single value. It sequentially goes through the array by using the result from the last operation as the basis for the next one.
Imagine you want to find the sum of an array of numbers:
const numbers = [1, 2, 3, 4, 5];
const sum = numbers.reduce((acc, num) => acc + num, 0);
console.log(sum);
// Result: 15
We have just added up all the numbers in the array and reduced it to a single value, which is the sum of all the numbers.
These methods are the bread and butter of handling arrays in JavaScript. Used correctly, they can drastically cut down on the amount of code written, making your code cleaner and more efficient.
Advanced JavaScript Array Methods
Welcome to the world of advanced JavaScript array methods! Here, we delve deeper into powerful methods that can help you manipulate arrays in exceptional ways. Mastering these methods not only boosts your programming skills but also provides you with the tricks to effectively solve complex code puzzles.
The find() method - the seeker
The find method, as its name implies, is a valuable tool for seeking out🕵️♀️ specific elements in an array. It executes a provided function on every element within the array until it finds one that meets the criteria. Once found, it stops and returns the value of the first element that passes the condition; if not, it yields undefined.
Here's an illustrative example:
const numbers = [4, 9, 16, 25, 29];
const first = numbers.find(num => num > 10);
console.log(first);
// Outputs: 16
The sort() method - the organizer
Sorting arrays is commonplace in programming, and JavaScript has a handy method for this very purpose. The sort method, without parameters, converts each element to a string and sorts the array in dictionary order (i.e., lexicographically). But it can also take a function as an argument to determine the sorting order.
Here's a guide on performing ascending and descending sorts:
- For ascending sort:
const numbers = [29, 16, 9, 4, 25];
numbers.sort((a, b) => a - b);
console.log(numbers); // Output: [4, 9, 16, 25, 29]
- For descending sort:
const numbers = [29, 16, 9, 4, 25];
numbers.sort((a, b) => b - a);
console.log(numbers); // Output: [29, 25, 16, 9, 4]
The indexOf() and splice() methods - the removers
Unleash the power of removal with indexOf()
and splice()
. These methods allow you to locate and remove elements from your array. Suppose you have an array of fruits and want to remove 'apple':
const fruits = ['banana', 'apple', 'orange'];
const index = fruits.indexOf('apple');
// Check if 'apple' is found (index is not -1)
if (index !== -1) {
// Remove 1 element at the index where 'apple' was found
fruits.splice(index, 1);
}
console.log(fruits);
// Output: ['banana', 'orange']
The slice() method - the cutter
Precision is key when you need a portion of your array. Enter the slice()
method, allowing you to extract a subarray without modifying the original. Extract the first two elements from an array:
const colors = ['red', 'blue', 'green', 'yellow'];
const slicedColors = colors.slice(0, 2);
console.log(slicedColors);
// Result: ['red', 'blue']
The slice(0, 2)
call means "start at index 0 and go up to (but not including) index 2". Therefore, it extracts elements at index 0 and index 1 from the colors
array, which are 'red'
and 'blue'
. As a result, slicedColors
contains ['red', 'blue']
.
The concat() method - the unifier
Achieve unity effortlessly with concat()
. Merge two arrays seamlessly:
const array1 = [1, 2, 3];
const array2 = [4, 5, 6];
const mergedArray = array1.concat(array2);
console.log(mergedArray);
// Result: [1, 2, 3, 4, 5, 6]
The reverse() method - the time traveler
Take a trip through time with reverse()
. This method reverses the order of your array, offering a new perspective.
Reverse an array of letters:
const letters = ['a', 'b', 'c', 'd'];
const reversedLetters = letters.reverse();
console.log(reversedLetters);
// Result: ['d', 'c', 'b', 'a']
And there we have it! These methods promise more efficiency with JavaScript arrays!
ES5 Syntax and ES6 Syntax for Array methods
JavaScript has always been robust in dealing with arrays, offering various methods to manipulate and handle array data efficiently. Over the years, JavaScript has evolved, and this evolution brought changes in syntax. The ES5 and ES6 are versions of the ECMAScript standard, which saw significant enhancements in array methods syntax.
Introduction to ES5 syntax
JavaScript ES5 brought a simplified way of interacting with arrays. Robust methods like "forEach", "map", "filter", "reduce", "find", and "sort"
were introduced.
Take an example of the “forEach” array method:
const array = [1, 2, 3];
array.forEach(function(element) {
console.log(element);
});
Here, the "forEach" method iterates over the array elements and logs each element to the console. This syntax is concise and straightforward, improving code readability and maintainability.
Introduction to ES6 syntax
ES6 or ES2015, introduced a more modern JavaScript version, with modified syntax for more clear and efficient code. One significant change was the introduction of Arrow Functions, a new way to define functions in a concise manner.
Using the “forEach” method in ES6:
const array = [1, 2, 3];
array.forEach(element => console.log(element));
In this case, we see how an arrow function streamlines the syntax further, making it more concise and simple to read.
How to use array methods in both ES5 and ES6 syntax?
In both ES5 and ES6, the array methods are accessed using dot notation followed by the method name. Here's how to use some common array methods in both syntaxes:
forEach
ES5: array.forEach(function(element) { console.log(element); });
ES6: array.forEach(element => console.log(element));
map
ES5: const newArray = array.map(function(element) { return element * 2; });
ES6: const newArray = array.map(element => element * 2);
filter
ES5: constfilteredArray = array.filter(function(element) { return element > 2; });
ES6: const filteredArray = array.filter(element => element > 2);
The array methods in JavaScript are potent tools for any developer, providing a faster and more efficient way of handling array operations. The updated syntax introduced in ES6 makes these methods even more powerful, and easier to use and understand.
Tips and tricks for optimizing Array Methods
First, utilize the power of chaining. JavaScript Array methods like 'map', 'filter', 'reduce', etc. can be elegantly chained together to perform complex operations in a single line. This not only makes your code cleaner and more readable but also improves its runtime efficiency.
Second, don’t forget about 'forEach'. While it's tempting to rely solely on 'map' and 'reduce' due to their powerful capabilities, 'forEach' can often be a simpler and more efficient solution for straightforward iterations through the array.
Best practices for using Array methods in performance-critical scenarios
When processing large arrays, consider using 'reduce'. It’s designed to produce a single output from a list of items, meaning it doesn't need to allocate memory for a new array thus making it highly memory efficient.
Experiment with ES5 and ES6 syntax, as the new ES6 syntax can often lead to cleaner, more efficient code. For instance, ES6 introduced arrow functions, which are more concise and solve several tricky issues with the 'this' keyword.
In the world of JavaScript arrays, always remember that the most efficient code isn't just about speed - it's about readability, memory efficiency, and maintainability as well.
Conclusion
Wrapping up our comprehensive guide on JavaScript array methods, let's take a moment to review the central topics we've explored. It's all about understanding these methods, their relevance, and how you can bolster your coding skills by mastering them.
Importance of understanding and mastering array methods in JavaScript programming
The array methods in JavaScript represent a rich toolset, facilitating the manipulation, transformation, search, and sorting of array elements in your code. Having a robust grasp on these concepts can tremendously enhance your programming skills, especially in JavaScript.
Moreover, mastering the different array syntax, namely ES5 and ES6, is equally valuable. It not only allows you to confidently traverse through the old and new scripts but also arms you with a broader knowledge base, helping you become a more proficient, agile, and adaptable programmer.
Final Thoughts 😎
When it comes to JavaScript array methods, understanding the basics and then venturing into advanced techniques and methods is key. Remember that practice makes perfect. So, keep exploring these methods, write code, make mistakes, learn, and grow. Remember, arrays are not just a series of items; they are the stepping stones to a more dynamic and interactive JavaScript journey!
We at CreoWis believe in sharing knowledge publicly to help the developer community grow. Let’s collaborate, ideate, and craft passion to deliver awe-inspiring product experiences to the world.
Let's connect:
This article is crafted by Chhakuli Zingare, a passionate developer at CreoWis. You can reach out to her on X/Twitter, LinkedIn, and follow her work on the GitHub.