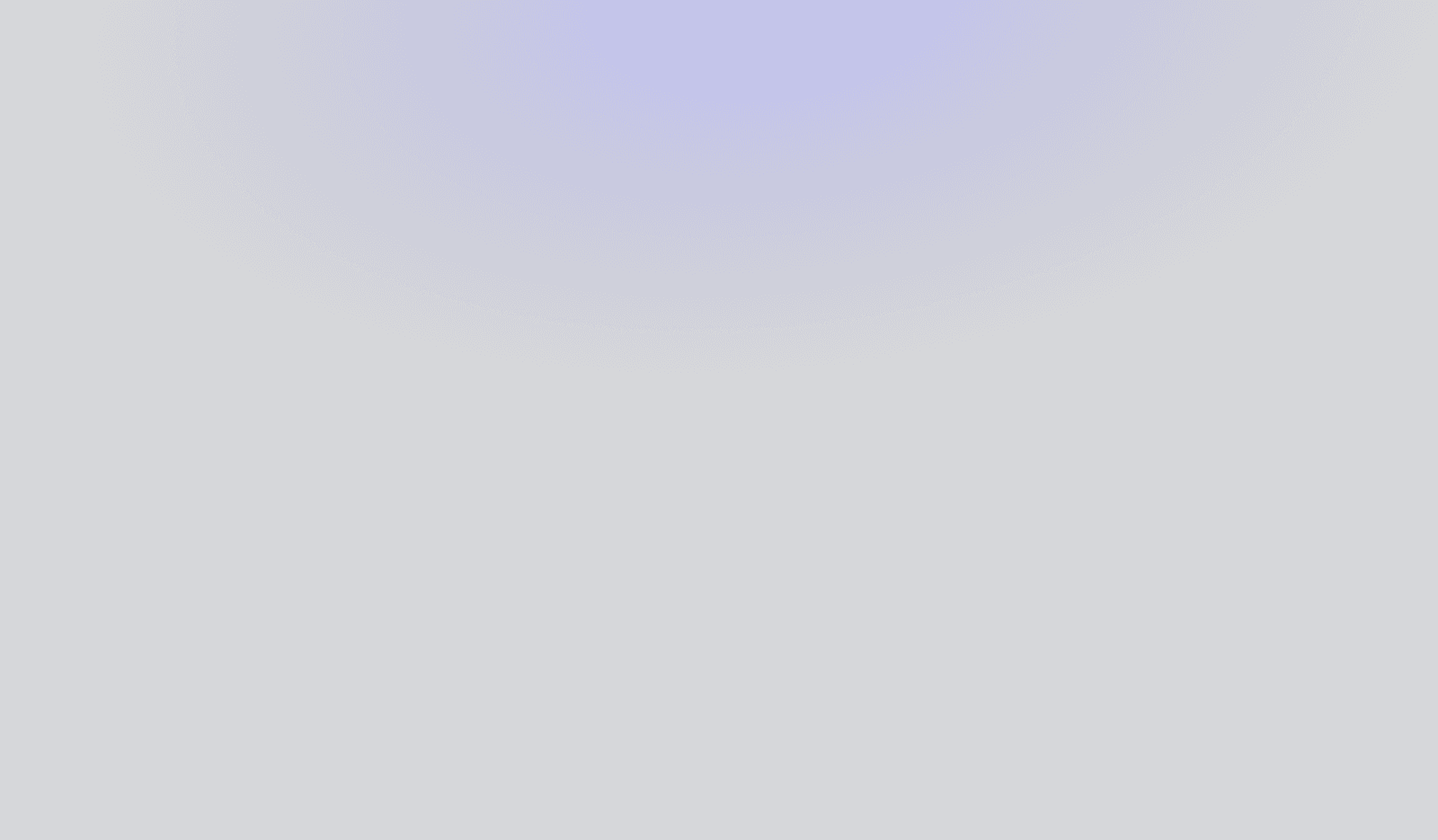
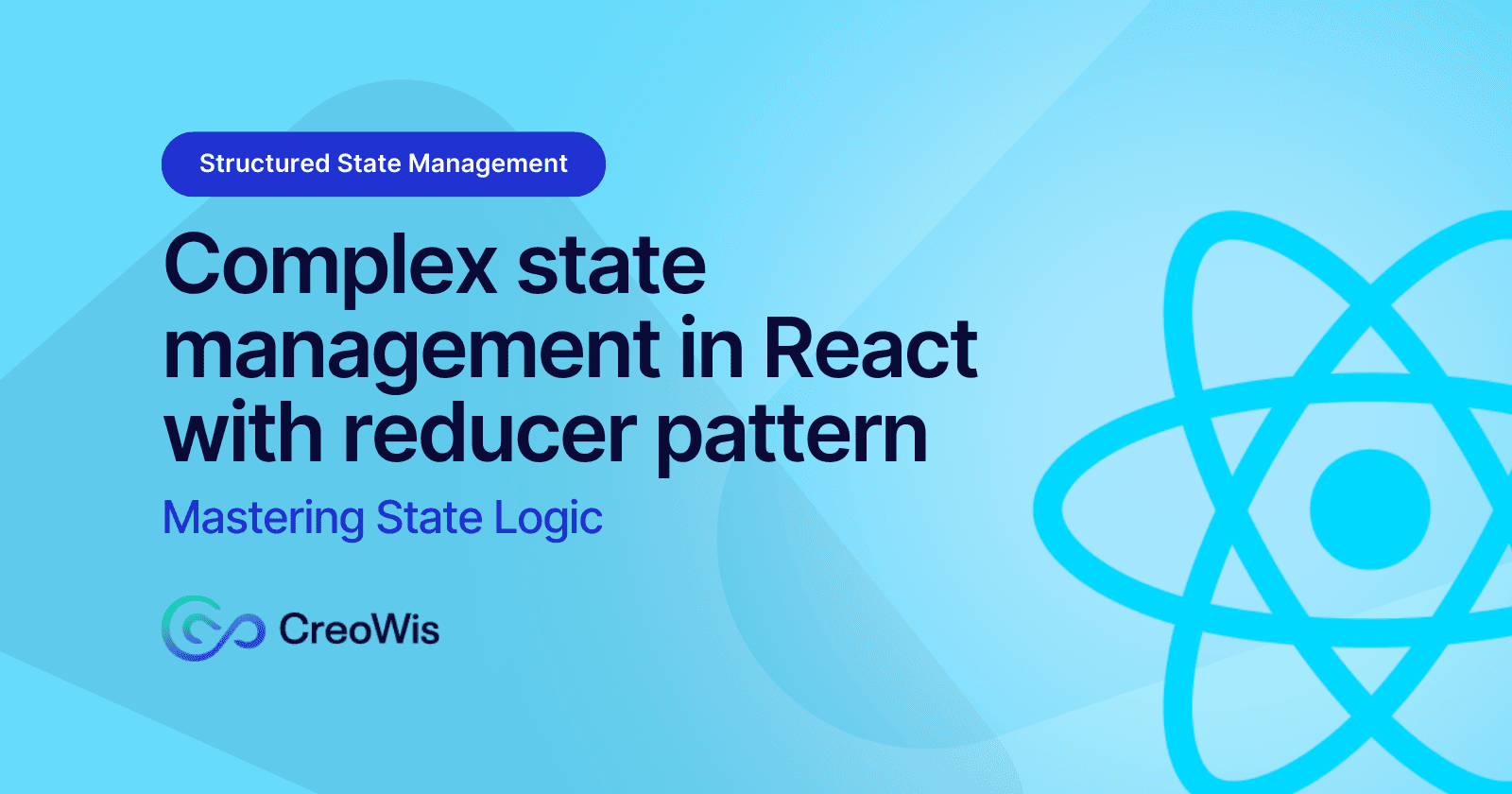
Complex state management in React with reducer pattern
Mastering predictable and scalable state handling in React with React's Reducer Pattern
Alright, React developers, buckle up! You have undoubtedly worked with useState
if you have experience with React. This hook is ideal for small, straightforward applications and is the standard for handling state in functional components. Declaring and updating state variables is made simple and uncomplicated with its help. But depending only on useState
can cause some issues when your application expands and the state gets more complicated.
Why useState
Can Become Challenging
UseState may be restrictive when your application grows for the following reasons:
Multiple State Variables: You will have multiple useState calls if your component contains a large number of state variables (for instance, a form with multiple fields). The component may become disorganized and more difficult to maintain as a result.
Complex State Transitions: UseState might result in repetitious and error-prone code as your state logic gets more intricate (for example, handling several related actions or updating the state based on the previous state). Tracking state management with useState alone can be challenging, particularly when nested state updates are involved.
State Duplication: You may find yourself repeating logic across several components in larger apps. Managing your state in several locations can easily become too much to handle.
Understanding the Reducer Pattern
Now useReducer enters the picture. It offers a more structured and expandable method of state management, particularly when state logic gets more intricate. UseReducer is used for state management when state updates need intricate logic or when the state is updated by numerous actions.
You can handle complicated state transitions in a more predictable and maintainable manner by centralizing state management using useReducer.
What Exactly is useReducer
?
At a high level, useReducer
is similar to useState
, but instead of directly updating the state, it uses a reducer function to describe how the state should change based on specific actions. The reducer is like a manager that takes in an action and updates the state accordingly.
Here's the structure:
Reducer Function: This defines how the state should change based on the action dispatched.
Initial State: The starting point for your state.
Dispatch: A function used to send actions to the reducer, triggering the state change
Example of Using useReducer
Let’s go through a simple counter-example to illustrate how useReducer
works.
import React, { useReducer } from "react";
const initialState = { count: 0 };
const reducer = (state, action) => {
switch (action.type) {
case "increment":
return { count: state.count + 1 };
case "decrement":
return { count: state.count - 1 };
case "reset":
return initialState;
default:
throw new Error("Unknown action type");
}
}
const Counter = () => {
const [state, dispatch] = useReducer(reducer, initialState);
return (
<div>
<p>Count: {state.count}</p>
<button onClick={() => dispatch({ type: "increment" })}>+</button>
<button onClick={() => dispatch({ type: "decrement" })}>-</button>
<button onClick={() => dispatch({ type: "reset" })}>Reset</button>
</div>
);
}
export default Counter;
Explanation:
Initial State:
{ count: 0 }
Reducer Function: Handles three actions:
"increment"
→ Adds 1 tocount
"decrement"
→ Subtracts 1 fromcount
"reset"
→ Resetscount
to 0
Dispatching Actions: Clicking a button sends an action to update the state.
Comparing useState
and useReducer
Let’s shift gears and dive into a scenario of comparing useState
and useReducer
with an example:
Using useState
for Multiple Form Fields:
First, let’s tackle the form using useState
for each individual field. Here’s what it might look like:
import React, { useState } from 'react';
const Form = () => {
const [name, setName] = useState('');
const [email, setEmail] = useState('');
const [password, setPassword] = useState('');
const handleSubmit = (e) => {
e.preventDefault();
console.log('Form Submitted', { name, email, password });
};
return (
<form onSubmit={handleSubmit}>
<input
type="text"
placeholder="Name"
value={name}
onChange={(e) => setName(e.target.value)}
/>
<input
type="email"
placeholder="Email"
value={email}
onChange={(e) => setEmail(e.target.value)}
/>
<input
type="password"
placeholder="Password"
value={password}
onChange={(e) => setPassword(e.target.value)}
/>
<button type="submit">Submit</button>
</form>
);
}
export default Form;
Why this might be problematic:
Lots of Repetition: If you have more fields to manage or if the form becomes more sophisticated, you wind up repeating the same useState pattern for every field, which can be inconvenient.
Harder to scale: Complex validation, conditionally turning off the submit button, and resetting the form fields all require code that is dispersed throughout the component and is more difficult to maintain.
Using useReducer
for a Multiple Form Fields:
Now, let's refactor the same form using useReducer
. With useReducer
, we can centralize the form state logic in one place and handle updates in a more organized manner.
import React, { useReducer } from 'react';
const initialState = { name: '', email: '', password: '' };
function formReducer(state, action) {
switch (action.type) {
case 'UPDATE_FIELD':
return { ...state, [action.field]: action.value };
case 'RESET':
return { ...initialState };
default:
return state;
}
}
const Form = () => {
const [state, dispatch] = useReducer(formReducer, initialState);
const handleChange = (e) => {
dispatch({
type: 'UPDATE_FIELD',
field: e.target.name,
value: e.target.value,
});
};
const handleSubmit = (e) => {
e.preventDefault();
console.log('Form Submitted', state);
};
const handleReset = () => {
dispatch({ type: 'RESET' });
};
return (
<form onSubmit={handleSubmit}>
<input
type="text"
name="name"
placeholder="Name"
value={state.name}
onChange={handleChange}
/>
<input
type="email"
name="email"
placeholder="Email"
value={state.email}
onChange={handleChange}
/>
<input
type="password"
name="password"
placeholder="Password"
value={state.password}
onChange={handleChange}
/>
<button type="submit">Submit</button>
<button type="button" onClick={handleReset}>Reset</button>
</form>
);
}
export default Form;
Why does useReducer
shine here:
Centralized State Management: All the form fields are handled in a single object.
Easier Field Updates: The
handleChange
function is much more scalable. You don’t need separate functions for every field. Instead, onehandleChange
can update any field in the form, making it super easy to add new fields without modifying the logic elsewhere.Action-Based Updates: The
dispatch
function and actions (e.g.,UPDATE_FIELD
,RESET
) clearly define how the state changes. It makes it easier to see exactly what’s happening when an action is triggered. Plus, as the form grows, you can add more actions (e.g., form validation, enabling/disabling buttons) in a structured way.State Reset: A common requirement in forms is to reset all fields. With
useReducer
, a simpleRESET
action handles this. WithuseState
, resetting each field separately would require a lot more boilerplate code.Easier to Scale: As the form grows, you can add new fields easily by just adding them to the
initialState
and modifying theformReducer
. You don’t need to worry about creating new state variables and state-setting functions for each new input.
Conclusion:
Why useReducer
is a Great Choice
Cleaner and More Maintainable Code: useReducer centralizes your state management, as was previously described. Everything is managed in one location rather to having several useState hooks dispersed across your component.
Enhanced Predictability: Since each action has a distinct kind, state transitions are simpler to monitor and comprehend. Larger applications that may need to manage several state changes in response to various user actions will find it very helpful.
Easier to Scale: The complexity of your state management increases with the size of your application. Your state logic will remain well-organized and isolated from the rest of the UI code thanks to
useReducer
. It becomes simple to add new actions or change preexisting logic.Less Repetitive Code: Using
useState
to manage complex states may require you to write different state setters for every section of the state, which would lead to code duplication.useReducer
keeps your code DRY (Don't Repeat Yourself) by requiring you to define only the reducer and dispatch actions.
When to Use useReducer
useReducer
excels when your app's state logic gets more complicated, whereas useState
is ideal for straightforward state management in React. useReducer
is the best option if you're dealing with several interconnected bits of state, need to manage different kinds of updates, or just want a simpler and easier way to manage state. It centralizes your state logic, makes the code easier to comprehend, and facilitates long-term app scaling.
Try using useReducer
in your next React project to see how it improves the predictability and maintainability of your state management.
Happy coding!
We at CreoWis believe in sharing knowledge publicly to help the developer community grow. Let’s collaborate, ideate, and craft passion to deliver awe-inspiring product experiences to the world.
Let's connect:
This article is crafted by Prachi Sahu, a passionate developer at CreoWis. You can reach out to her on X/Twitter, LinkedIn, and follow her work on GitHub.